User Onboarding to New Chains & Wallets
Many applications try to create user accounts to improve the user experience. Some common use cases include applications deploying embedded wallets for users, DeFi platforms onboarding users into vaulted accounts, and applications deploying their own chains.
Decent streamlines user onboarding by enabling users to swap directly into the application's required token(s) and fill this application account.
- Developers can embed Decent's onboarding modal in account creation and profile pages.
- Developers can specify a target token (e.g., USDC on Arbitrum) and wallet address (e.g., a new Privy or Dynamic wallet) as the destination.
- Users can connect an existing EOA and view all of their token balances across chains.
- Users can select a target balance and enter an amount to transfer.
- In one click, users can onboard directly into their account!
Key supplementary links:
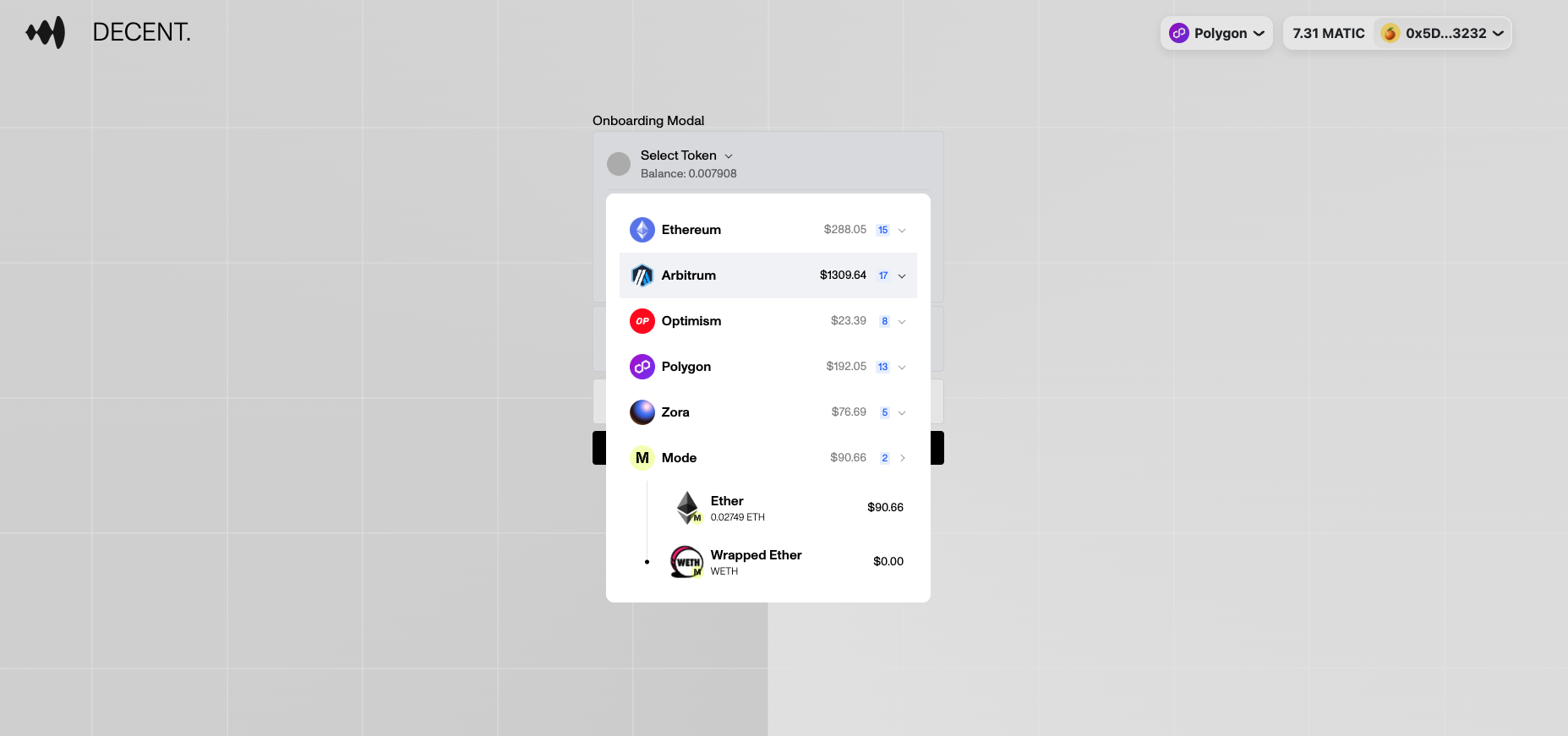
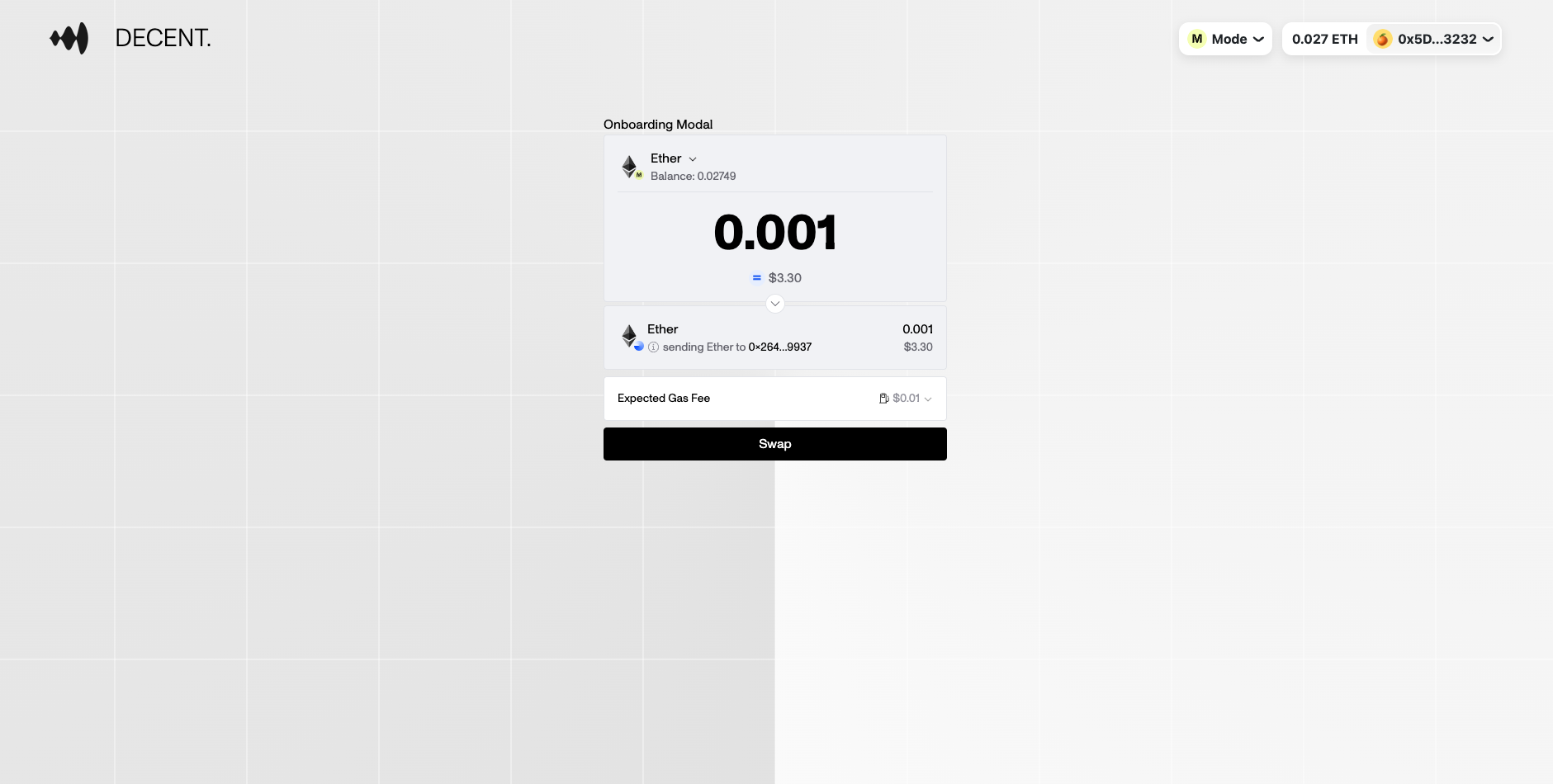
Component Props
Parameter | Description | Type (see examples below) | Required |
---|---|---|---|
apiKey | Target contract's chain ID. | ChainId | true |
wagmiConfig | Configuration for your wagmi client. | Config *imported from @wagmi/core | true |
onConnectWallet | Callback function to handle connecting wallets from the modal. | () => void | true |
chainIds | Optionally support only a subset of Decent chains. | ChainId[] | false |
className | Tailwind CSS styling. | string | false |
onDstTokenSelected | Callback function to update state based on dst token selection. | (t: TokenInfo) => void | false |
onSrcTokenSelected | Callback function to update state based on src token selection. | (t: TokenInfo) => void | false |
receiverAddress | Address to receive the swapped funds. Will default to connected address. | 0x${string} | false |
selectedSrcToken | Force specific source token. | SelectedChainOrToken | false |
selectedDstToken | Force specific destination token. | SelectedChainOrToken | false |
sendInfoTooltip | Text for the tooltip to explain destination for transaction. | string | false |
Sample Implementation
Below is an example of how to style and install the Onboarding Modal.
Please be sure to view the Getting Started section before progressing through these steps.
Step one (optional): Write a custom theme for the modal
Import the BoxThemeProvider
at the top of your component tree. You can either write your own custom theme or import Decent's darkMode
as an alternative styling option.
import "@decent.xyz/the-box/index.css";
import { BoxThemeProvider } from "@decent.xyz/the-box";
// Eligible theme elements that you may choose to customize.
export const onboardingDarkTheme = {
mainBgColor: '#121212',
mainTextColor: '#ffffff',
lighterBgColor: '#1B1B1B',
tokenSwapCardBgColor: '#1B1B1B',
buyBtnBgColor: '#8236FD',
buyBtnTextColor: '#ffffff',
switchBtnBgColor: '#3A3842',
tokenDialogHoverColor: '#444444',
boxSubtleColor1: '#605E6E',
borderColor: '#27252B',
loadShineColor1: '#121212',
loadShineColor2: '#333333',
greenBadgeTextColor: '#11BC91',
greenBadgeBgColor: '#123129',
yellowBadgeTextColor: '#FF8B31',
yellowBadgeBgColor: '#3D2818',
blueBadgeBgColor: '#256AF633',
blueBadgeTextColor: '#256AF6',
circleLinkChainColor: '#9969FF',
circleLinkBgColor: '#261D3C',
borderRadius: '0',
buyBtnBorderRadius: '999px',
}
...
function MyApp({ Component, pageProps }: AppProps) {
return (
<BoxThemeProvider theme={onboardingDarkTheme}>
<Component {...pageProps} />
</BoxThemeProvider>
);
};
Step two: Import the Onboarding Modal into the appropriate page or component
The Onboarding Modal requires a wagmiConfig
and handler to connect your wallet. Please visit this section of wagmi's docs to reference how you can use wagmi with popular third party wallet connection libraries like RainbowKit, ConnectKit, and others. Your wallet connection library will include an import similar to the useConnectModal
hook depicted here.
import { SwapModal } from "@decent.xyz/the-box";
import { useConnectModal } from "@rainbow-me/rainbowkit";
import { wagmiConfig } from '../your_wagmi_config_path';
...
const Component = () => {
const { openConnectModal } = useConnectModal();
return (
<OnboardingModal
receiverAddress=''
wagmiConfig={wagmiConfig}
apiKey={process.env.NEXT_PUBLIC_DECENT_API_KEY as string}
onConnectWallet={openConnectModal}
sendInfoTooltip={`You're fillling your application wallet!`}
className={''}
selectedDstToken={{
chainId: ChainId.BASE,
tokenInfo: getNativeTokenInfo(ChainId.BASE),
}}
chainIds={[
ChainId.ETHEREUM,
ChainId.ARBITRUM,
ChainId.OPTIMISM,
ChainId.POLYGON,
ChainId.ZORA,
ChainId.MODE,
]}
/>
);
};